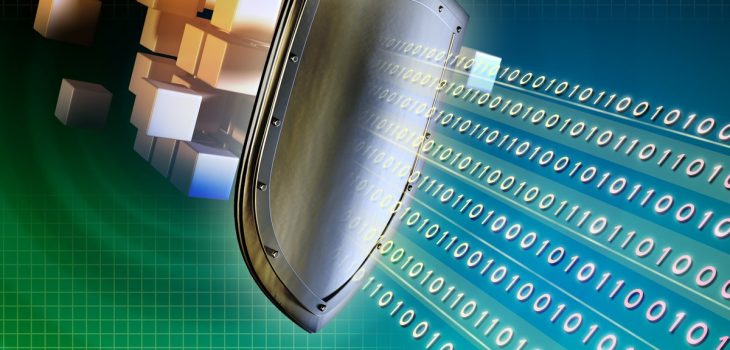
FieldShield SDK
IRI provides a software development kit (SDK) to help FieldShield users apply column-level encryption, decryption, hashing, and redaction algorithms in Java and .NET projects for more in-situ or dynamic data masking requirements. This article covers the library and the field/column-level data protection functions it supports.
Code for the examples shown is in C#, though the function calls will be the same in all the .NET languages. Java uses the same methods, but some require an extra parameter to be used; those cases are mentioned where needed.
There are two types of classes in the SDK: encryption classes and conversion classes. The encryption and decryption classes are:
- AES256
- ASCII
- Alphanumeric
The conversion classes are:
- Hexadecimal
- Base64
- Hash Sha256
- Character Replacing.
Encryption and Decryption Classes
<class type> obj= new <class type>();
int iResult = obj.begin();
obj.setPass(“TEST”);
string output = obj.doTransform(“TestString”);
obj.end();
- To use a FieldShield encryption class, create an encryption class object of the desired encryption type. The options are:
- enc_aes256
- enc_fp_ascii
- enc_fp_alphanum
(where fp refers to format-preserving encryption)
- Set up the object using the begin() method.
- Set a password for the encryption class using the setPass() method.
- Call the doTransform() method with a string parameter (the string to be encrypted). The return value is the new encrypted value.
- Once finished, delete the encryption class object with the end() method.
To use these functions in Java, you need an extra parameter for all of the function calls. The parameter is a pointer to the object which comes from a call to getptr().
Example begin() call in Java: obj.begin(obj.getptr());
To use the decryption value of the chosen encryption class, replace “enc” with “dec”. The rest of the method calls will be the same.
Example enc_aes256 becomes dec_aes256.
Conversion Classes
Hex / Base64 / Hash Sha256
<class type> obj = new <class type>();
string encoded = obj.<class encode/decode method>(inputString, input.Length);
Replace Characters
int[] position = {0,4};
replace_char obj = new replace_char();
string encoded = obj.maskString(inputString, ‘#’, position);
- To use a FieldShield conversion class, create a conversion class object of the desired conversion type. The options are:
- hex
- base64
- hash_sha256
- replace_char
- To encode/decode a value, call the chosen classes encode/decode method. This method needs two parameters; the string to encode/decode, and the length of the string to encode. The method returns the encoded/decoded string.
- hex_encode/hex_decode
- base64_encode/base64_decode
- sha256_hash
- maskString
- The sha256_hash method is static in .NET and is called from a static hash_sha256 object. In Java, it is called from an instance of a hash_sha256 object.
- maskString requires different parameters than the other methods. Three parameters are needed: the first is the string to replace the characters in; the second is the character to replace them with; and the third is a two-dimensional array containing the value of the position to start at and the length of characters to replace.
To use the hash_sha256 class in Java, you need an extra parameter for all of the function calls. The parameter is a pointer to the object which comes from a call to getptr().
Example sha256_hash() call in Java: obj.sha256_hash(obj.getptr(), input, input.length());
An example of encryption used in Java is shown below. This program retrieves the Phone_Number column from an Oracle database and encrypts it using FieldShield’s format-preserving alphanumeric encryption function.
The functions in the SDK are compatible with those in the main FieldShield package so you can encrypt in one and decrypt in another, for example. For any questions or technical support using the SDK, contact your IRI representative.